If you have two controls bound to the same datasource, and you do
not want them to share the same position, then you must make sure that the BindingContext
member of one control differs from the BindingContext member of the other control. If
they have the same BindingContext, they will share the same position in the
datasource.
If you add for example a ComboBox and a ListBox to a form, the
default behavior is for the BindingContext member of each of the two controls to be set
to the Form's BindingContext. Thus, the default behavior is for the ListBox and ComboBox
to share the same BindingContext, and hence the selection in the ComboBox is synchronized
with the current row of the ListBox. If you do not want this behavior, you should create
a new BindingContext member for at least one of the controls.
comboBox1.BindingContext
= new
BindingContext();
The following example shows, how you can you prevent
a ComboBox and ListBox bound to the same DataTable from sharing the same current
position.
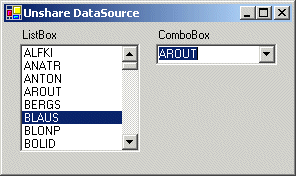
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Data.SqlClient;
namespace Akadia.UnshareDataSource
{
public class UnshareDataSource : System.Windows.Forms.Form
{
private System.Windows.Forms.ListBox listBox1;
private System.Windows.Forms.ComboBox comboBox1;
private System.Windows.Forms.Label lblListBox;
private System.Windows.Forms.Label lblComboBox;
private System.ComponentModel.Container
components = null;
public UnshareDataSource()
{
InitializeComponent();
}
....
....
// The main entry point for the
application.
[STAThread]
static void Main()
{
Application.Run(new UnshareDataSource());
}
private void UnshareDataSource_Load(
object sender, System.EventArgs e)
{
// Read Table customers into
DataSet
string ConnectionString =
"data source=xeon;uid=sa;password=manager;
database=northwind";
SqlConnection cn = new
SqlConnection(ConnectionString);
string sqlString = "SELECT * FROM customers";
SqlDataAdapter dataAdapter =
new SqlDataAdapter(sqlString, cn);
DataSet dataSet = new DataSet();
dataAdapter.Fill(dataSet, "customers");
// Attach dataset's DefaultView to
the combobox
listBox1.DataSource =
dataSet.Tables["customers"].DefaultView;
listBox1.DisplayMember = "CustomerID";
// Attach dataset's DefaultView to
the combobox
// Unshare the Datasource from the listBox1
comboBox1.BindingContext = new
BindingContext();
comboBox1.DataSource =
dataSet.Tables["customers"].DefaultView;
comboBox1.DisplayMember = "CustomerID";
}
}
}